Dynamsoft Developer Blog
Featured Content
View More >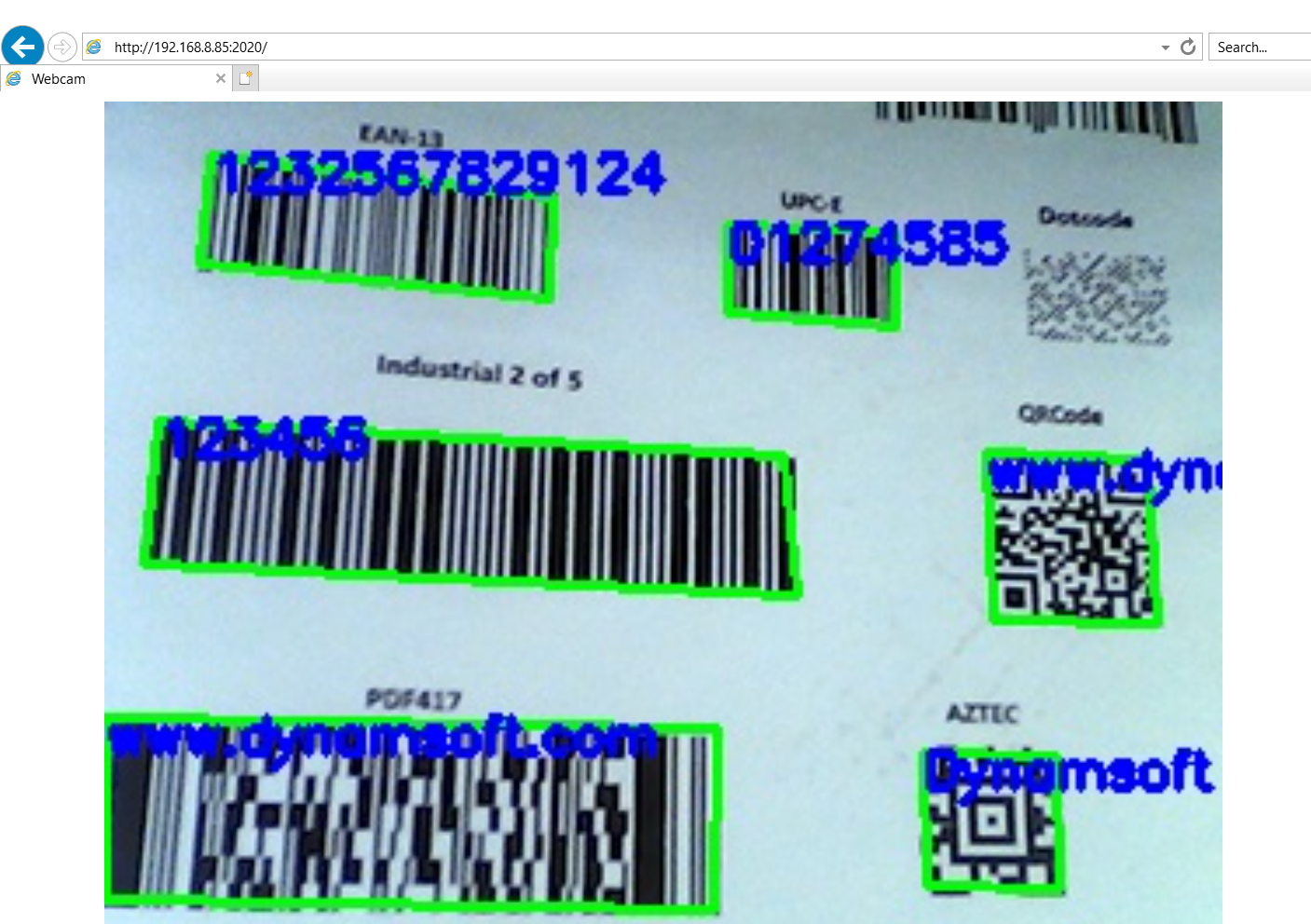
Building a Real-Time Barcode QR Code Scanner with Node.js for Desktop and Web
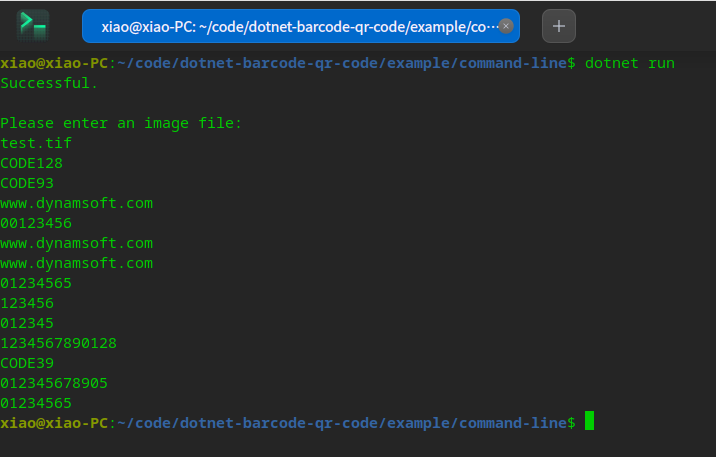
How to Build .NET 6 Barcode and QR Code SDK for Windows, Linux & macOS
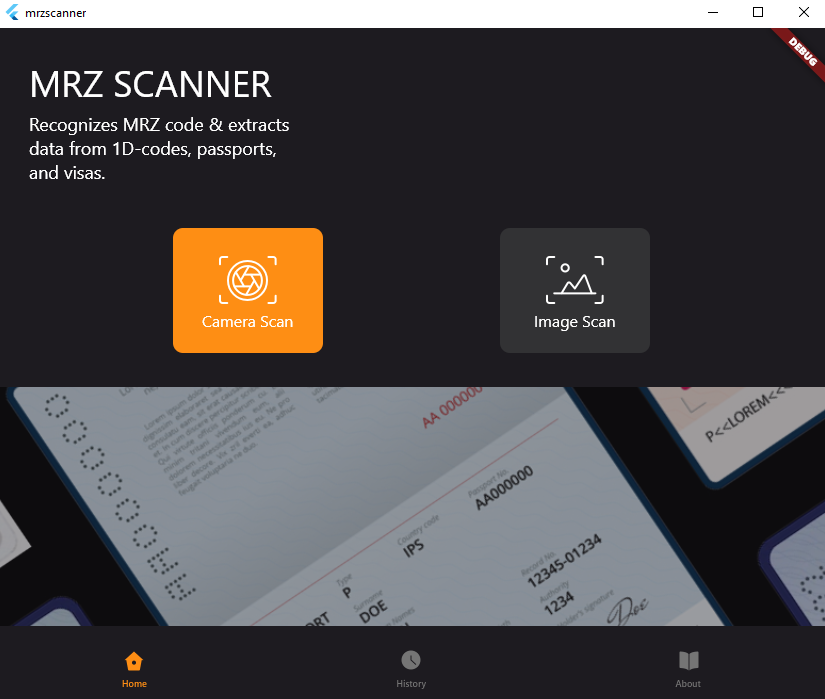
How to Create a Cross-platform MRZ Scanner App Using Flutter and Dynamsoft Label Recognizer
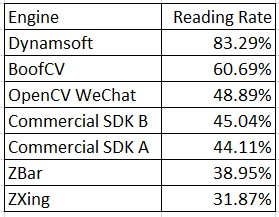
QR Code Reading Benchmark and Comparison
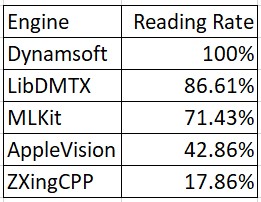
What are the Best Data Matrix Reading SDKs?